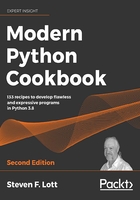
1 Numbers, Strings, and Tuples
This chapter will look at some of the central types of Python objects. We'll look at working with different kinds of numbers, working with strings, and using tuples. These are the simplest kinds of data Python works with. In later chapters, we'll look at data structures built on these foundations.
Most of these recipes assume a beginner's level of understanding of Python 3.8. We'll be looking at how we use the essential built-in types available in Python—numbers, strings, and tuples. Python has a rich variety of numbers, and two different pision operators, so we'll need to look closely at the choices available to us.
When working with strings, there are several common operations that are important. We'll explore some of the differences between bytes—as used by our OS files, and strings—as used by Python. We'll look at how we can exploit the full power of the Unicode character set.
In this chapter, we'll show the recipes as if we're working from the >>> prompt in interactive Python. This is sometimes called the read-eval-print loop (REPL). In later chapters, we'll change the style so it looks more like a script file. The goal in this chapter is to encourage interactive exploration because it's a great way to learn the language.
We'll cover these recipes to introduce basic Python data types:
- Working with large and small integers
- Choosing between float, decimal, and fraction
- Choosing between true pision and floor pision
- Rewriting an immutable string
- String parsing with regular expressions
- Building complex strings with f-strings
- Building complex strings from lists of characters
- Using the Unicode characters that aren't on our keyboards
- Encoding strings – creating ASCII and UTF-8 bytes
- Decoding bytes – how to get proper characters from some bytes
- Using tuples of items
- Using NamedTuples to simplify item access in tuples
We'll start with integers, work our way through strings, and end up working with simple combinations of objects in the form of tuples and NamedTuples.