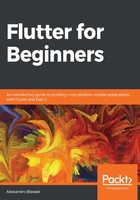
Functions
In Dart, Function is a type, like String or num. This means that they can also be assigned to fields or local variables or passed as parameters to other functions; consider the following example:
String sayHello() {
return "Hello world!";
}
void main() {
var sayHelloFunction = sayHello; // assigning the function
// to the variable
print(sayHelloFunction()); // prints Hello world!
}
In this example, the sayHelloFunction variable stores the sayHello function itself and does not invoke it. Later on, we can invoke it by adding () to the variable name just as though it was a function.
The function return type can be omitted as well, so the Dart analyzer infers the type from the return statement. If no return statement is provided, it assumes return null. If you want to tell it that it doesn't have a return, you should mark it as void:
sayHello() { // The return type stills String
return "Hello world!";
}
Another way to write this function is by using the shorthand syntax, () => expression;, which is also called the Arrow function or the Lambda function:
sayHello() => "Hello world!";
You cannot write statements in place of expression, but you can use the already known conditional expressions (that is, ?: or ??).