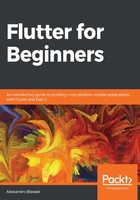
The enum type
The enum type is a common type used by most languages to represent a set of finite constant values. In Dart, it is no different. By using the enum keyword, followed by the constant values, you can define an enum type:
enum PersonType {
student, employee
}
Note that you define just the value names. enum types are special types with a set of finite values that have an index property representing its value. Now, let's see how it works.
First, we add a field to our previously defined Person class to store its type:
class Person {
...
PersonType type;
...
}
Then, we can use it just like any other field:
main() {
print(PersonType.values); // prints [PersonType.student,
//PersonType.employee]
Person somePerson = new Person();
somePerson.type = PersonType.employee;
print(somePerson.type); // prints PersonType.employee
print(somePerson.type.index); // prints 1
}
You can see that the index property is zero, based on the declaration position of the value.
Also, you can see that we are calling the values getter on the PersonType enum directly. This is a static member of the enum type that simply returns a list with all of its values. We will examine this further soon.