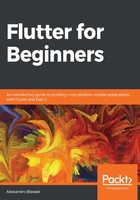
Static fields and methods
As you already know, fields are nothing more than variables that hold object values, and methods are simple functions that represent object actions. In some cases, you may want to share a value or method between all of the object instances of a class. For this use case, you can add the static modifier to them, as follows:
class Person {
// ... class fields definition
static String personLabel = "Person name:";
String get fullName => "$personLabel $firstName $lastName";
// modified to print the new static field "personLabel"
}
Hence, we can change the static field value directly on the class:
main() {
Person somePerson = Person("clark", "kent");
Person anotherPerson = Person("peter", "parker");
print(somePerson.fullName); // prints Person name: clark kent
print(anotherPerson.fullName); // prints Person name: peter park
Person.personLabel = "name:";
print(somePerson.fullName); // prints name: clark kent
print(anotherPerson.fullName); // prints name: peter parker
}
The static fields are associated with the class, rather than any object instance. The same goes for the static method definitions. We can add a static method to encapsulate the name printing, as demonstrated in the following code block, for example:
class Person {
// ... class fields definition
static String personLabel = "Person name:";
static void printsPerson(Person person) {
print("$personLabel ${person.firstName} ${person.lastName}");
}
}
Then, we can use this method to print a Person instance, just like we did before:
main() {
Person somePerson = Person("clark", "kent");
Person anotherPerson = Person("peter", "parker");
Person.personLabel = "name:";
Person.printsPerson(somePerson); // prints name: clark kent
Person.printsPerson(anotherPerson); // prints name: peter park
}
We could modify the fullName getter on the Person class to not use the personLabel static field, to make more sense and obtain distinct results according to our requirements:
class Person {
// ... class fields definition
String get fullName => "$firstName $lastName";
}
main() {
Person somePerson = Person("clark", "kent");
Person anotherPerson = Person("peter", "parker");
print(somePerson.fullName); // prints clark kent
print(anotherPerson.fullName); // prints peter parker
Person.printsPerson(somePerson); // prints Person name: clark kent
Person.printsPerson(anotherPerson); // prints Person name: peter park
}
As you can see, static fields and methods allow us to add specific behaviors to classes in general.