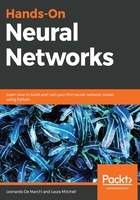
Feature engineering in Keras
Keras provides a nice and simple interface to do feature engineering. A task that we will study in particular in this book is image classification. For this task, Keras provides the ImageDataGenerator class, which allows us to easily pre-process and augment the data.
The augmentation we are going to perform is aimed at generating more images using some random transformations such as zooming, flipping, shearing, and shifting. These transformations help prevent overfitting and make the model more robust to different image conditions, such as brightness.
We will see the code first and then explain what it does. Following Keras' documentation (https://keras.io/), it's possible to create a generator with the mentioned transformations with the code:
from keras.preprocessing.image import ImageDataGenerator datagen = ImageDataGenerator( rotation_range=45, width_shift_range=0.25, height_shift_range=0.25, rescale=1./255, shear_range=0.3, zoom_range=0.3, horizontal_flip=True, fill_mode='nearest')
For the generator, it's possible to set a few parameters:
- The rotation_range parameter represents value in degrees (0-180), which will be used to randomly find a value to rotate the inputs.
- width_shift and height_shift are ranges (as a fraction of total width or height) within which it randomly translates pictures vertically or horizontally.
- Scale is a common operation used to re-scale a raw image. In this case, we have RGB images, in which each pixel is represented by a value between 0 and 255. Because of this, we use a scaling factor of 1/255 so our values now will be between 0 and 1. We do this as otherwise the numbers would be too high given the typical learning rate, one of the parameters of our network.
- shear_range is used for randomly applying shearing transformations.
- zoom_range is used to create additional pictures by randomly zooming inside pictures.
- horizontal_flip is a Boolean value used to create additional pictures by randomly flipping half of the image horizontally. This is useful when there are no assumptions of horizontal asymmetry.
- fill_model is the strategy used for filling in new components
In this way, from one image, we can create many to feed to our model. Notice that we only initialized the object so far, so no instruction has being executed as the generator will perform the action only when it's called; it will be done later on.