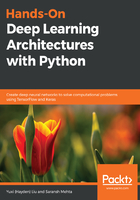
上QQ阅读APP看书,第一时间看更新
Building a graph
A TensorFlow graph is a series of operations organized in graph. The model architecture is first built in the form of a TensorFlow graph. You need to keep in mind three basic operations:
- tf.constant: Holds a constant tensor just like a constant in Python, but unlike Python, it gets activated only during a TensorFlow Session.
- tf.Variable: Holds a variable tensor that is learnable during training and updates value.
- tf.Placeholder: This is an interesting feature of TensorFlow. At the time of building the graph, we don't provide the input data. But, it is required to lay out the shape and data type of input that the graph will be receiving. Thus, placeholder acts as a container that will allow the flow of input tensors when a Session is activated.
Let's try to add two constants in TensorFlow as follows:
>>>import tensorflow as tf
>>>t1 = tf.constant('hey')
>>>t2 = tf.constant('there')
>>sum = t1 + t2
>>>print(sum)
This will output something like this: add:0, shape=(), dtype=string. You were expecting heythere? This doesn't happen because TensorFlow runs the graph only when a Session is activated. By defining the constants, we just made a graph, and that's why the print was trying to tell what the sum would be when the graph is run. So, let's create a Session.