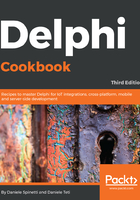
上QQ阅读APP看书,第一时间看更新
There's more...
Streams are very powerful and their uniform interface helps us write portable and generic code. With the help of streams and polymorphism, we can write code that uses TStream to do some work, without knowing what kind of stream it is!
Also, a well-known possibility is that if you ever need to write a program that needs to access the good old TD_INPUT, STD_OUTPUT, or STD_ERROR, you can use THandleStream to wrap these system handles with a nice TStream interface using the following code:
program StdInputOutputError; //the following directive instructs the compiler to create a //console application and not a GUI one, which is the default. {$APPTYPE CONSOLE} uses System.Classes, // required for Stream classes Winapi.Windows; // required to have access to the STD_* handles var StdInput: TStreamReader; StdOutput, StrError: TStreamWriter; begin StdInput := TStreamReader.Create( THandleStream.Create(STD_INPUT_HANDLE)); StdInput.OwnStream; StdOutput := TStreamWriter.Create( THandleStream.Create(STD_OUTPUT_HANDLE)); StdOutput.OwnStream; StdError := TStreamWriter.Create( THandleStream.Create(STD_ERROR_HANDLE)); StdError.OwnStream; { HERE WE CAN USE OURS STREAMS } // Let's copy a line of text from STD_IN to STD_OUT StdOutput.writeln(StdInput.ReadLine); { END - HERE WE CAN USE OURS STREAMS } StdError.Free; StdOutput.Free; StdInput.Free; end;
Moreover, when you work with file-related streams, the TFile class (contained in System.IOUtils.pas) is very useful, and has some helper methods to write shorter and more readable code.