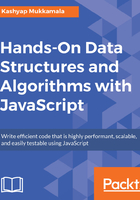
Final application logic
So, now our application is ready to go. We have added the logic to stack the states that are being navigated to and we also have the logic for when the user hits the Back button. When we put all this logic together in our app.component.ts, we have the following:
import {Component, ViewEncapsulation} from '@angular/core';
import {Router, NavigationEnd} from '@angular/router';
import {Stack} from "./utils/stack";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss', './theme.scss'],
encapsulation: ViewEncapsulation.None
})
export class AppComponent {
constructor(private stack: Stack, private router: Router) {
this.router.events.subscribe((val) => {
if(val instanceof NavigationEnd) {
this.stack.push(val);
}
});
}
goBack() {
let current = this.stack.pop();
let prev = this.stack.peek();
if (prev) {
this.stack.pop();
this.router.navigateByUrl(prev.urlAfterRedirects);
} else {
this.stack.push(current);
}
}
}
We also have some supplementary stylesheets used in the application. These are obvious based on your application and the overall branding of your product; in this case, we are going with something very simple.
For the AppComponent styling, we can add component-specific styles in app.component.scss:
.active {
color: red !important;
}
For the overall theme of the application, we add styles to the theme.scss file:
@import '~@angular/material/theming';
// Plus imports for other components in your app.
// Include the common styles for Angular Material. We include this here so that you only
// have to load a single css file for Angular Material in your app.
// Be sure that you only ever include this mixin once!
@include mat-core();
// Define the palettes for your theme using the Material Design palettes available in palette.scss
// (imported above). For each palette, you can optionally specify a default, lighter, and darker
// hue.
$candy-app-primary: mat-palette($mat-indigo);
$candy-app-accent: mat-palette($mat-pink, A200, A100, A400);
// The warn palette is optional (defaults to red).
$candy-app-warn: mat-palette($mat-red);
// Create the theme object (a Sass map containing all of the palettes).
$candy-app-theme: mat-light-theme($candy-app-primary, $candy-app-accent, $candy-app-warn);
// Include theme styles for core and each component used in your app.
// Alternatively, you can import and @include the theme mixins for each component
// that you are using.
@include angular-material-theme($candy-app-theme);
This preceding theme file is taken from the Angular material design documentation and can be changed as per your application's color scheme.
Once we are ready with all our changes, we can run our application by running the following command from the root folder of our application:
ng serve
That should spin up the application, which can be accessed at http://localhost:4200.
From the preceding screenshot, we can see that the application is up-and-running, and we can navigate between the different states using the Back button we just created.