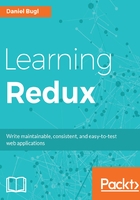
上QQ阅读APP看书,第一时间看更新
Updating the state
Finally, we define the tick() method we referenced earlier. In this method, we will update the state by increasing seconds by one. React provides the this.setState(state) method, which works similarly to using spread syntax to update objects. Only the properties passed to this.setState will be updated/overwritten. For example, if we consider this snippet:
// in constructor() method: this.state = { seconds: 0, somethingElse: true } // in tick() method: this.setState({ seconds: this.state + 1 })
The resulting state will be as follows:
{ seconds: 1, somethingElse: true }
It works similar to the following code using the spread operator:
const state = { seconds: 0, somethingElse: true } const newState = { seconds: state.seconds + 1 } const resultingState = { ...state, ...newState }
Now that we understand how updating state in React works, we can write our tick() method:
tick () { const { seconds } = this.state this.setState({ seconds: seconds + 1 }) }
Do not update the state by writing to this.state directly—your component will not be re-rendered!