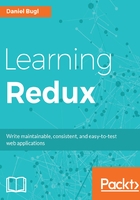
Setting up webpack
Now, we will install webpack, which will be used to build your project. Webpack is a module bundler. It is used to bundle, transform, and package various files to be used in a browser. This includes JavaScript, JSX, HTML, and assets.
The advantages of using a module bundler, such as webpack, are listed as follows:
It can bundle various kinds of JavaScript modules: ES Modules, CommonJS, and AMD.
It works together with Babel to transpile newer JavaScript syntax, such as ES2015.
Not just JavaScript, but various files can be preprocessed during compilation. For example, images can be converted to base64, or template files can be compiled into HTML.
It can create a single bundle or multiple chunks. Splitting your code into multiple chunks can reduce initial loading time, by, for example, only loading certain code when it is needed.
It resolves dependencies during compilation and removes unused code, thus reducing the code file size.
It is extremely extensible and has a rich ecosystem with many plugins already available.
Webpack can be installed via npm. We will also install webpack-dev-server, which can run our project on a web server and provide live reloading; live reloading means that when files change, webpack automatically rebuilds the project and refreshes the website in your browser:
We start by installing webpack and webpack-dev-server:
npm install --save-dev webpack webpack-dev-server
Next, we will need to create a configuration file for webpack. This file is called webpack.config.js and contains the following code:
const path = require('path')
module.exports = {
entry: './src/index.js',
output: {
path: path.resolve('dist'),
filename: 'main.js'
},
This configuration tells webpack where the entry and output files for our project are.
Now, we need to define loaders. Loaders tell webpack what to do with the entry file(s). Since we will be using Babel to transpile our JavaScript files, we will define the babel-loader for .js(x) files:
module: {
loaders: [
{ test: /.jsx?$/, loader: 'babel-loader', exclude:
/node_modules/
}
]
}
}
That's all for the webpack configuration for now. If we want to find out more about webpack, we can check out its documentation at https://webpack.js.org/configuration/.