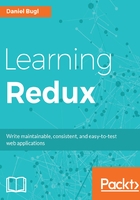
ES2015 - arrow functions
There is a new, more concise way to define functions in ES2015, called arrow function syntax. We could write the createPost(user, text) function in arrow function syntax, as follows:
const createPost = (user, text) => {
return {
type: CREATE_POST,
user: user,
text: text
}
}
Additionally, ES2015 allows us to shorten property definitions where the object key and constant name are the same (for example, user: user):
const createPost = (user, text) => {
return { type: CREATE_POST, user, text }
}
And we could return the object directly, by wrapping it with ( ) brackets:
const createPost = (user, text) => ({ type: CREATE_POST, user, text })
The format that you use is mostly of your preference. However, there is one key difference between arrow functions and normal functions that you should be aware of: Arrow functions do not have their own this context; they inherit it from the parent. As a result, you do not need to write var that = this or var _this = this anymore. You can simply use an arrow function because it does not overwrite this.