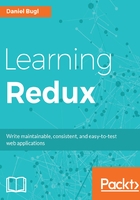
Implementing reducers
In the first chapter, we learned about reducers, functions that take the current state and an action and return the new state of the application. As you now know, actions only describe what happened. Reducers use that information to compute the new state.
To be able to figure out state changes, we should first think about the state of our application. We have already done this in the first chapter, and our state looks as follows:
{
posts: [
{ user: 'dan', category: 'hello', text: 'Hello World!' },
{ user: 'des', category: 'welcome', text: 'Welcome to the blog' }
],
filter: 'hello'
}
As you can see, we have two separate sub-states in the main state object: posts and filter. This is a good hint for making two reducers: a postsReducer to handle the posts sub-state, and a filterReducer to handle the filter sub-state.
If we wanted to add more features, such as showing user information, we would make a new users sub-state, which stores all users. A separate usersReducer would then handle all actions related to that data set.
There are no generic rules on how to split up reducers and your application state. It depends on your projects' requirements and the existing structure. As a good rule of thumb, try not to make one reducer that handles all the actions, but also do not make a separate reducer for each action. Group them in a way that makes sense for the application structure. Throughout the following chapters, we will focus on how to structure a growing application in practice.