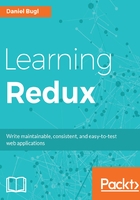
Combining reducers
Now, all that is left to do is combining these two reducers together to handle the whole state and all actions of the application:
function appReducer (state = {}, action) {
return {
posts: postsReducer(state.posts, action),
filter: filterReducer(state.filter, action),
}
}
The default state for our app is an empty object, {}. We create the state object by passing the substates and actions down to our other reducers. On initialization, these reducers get passed undefined, because state.posts and state.filter do not exist, yet. As a result, the default state defined in the subreducers will be used.
Since an appReducer that combines other reducers is such a common function to write, Redux provides a combineReducers helper function, which creates a function that essentially does the same thing as the appReducer function we defined earlier:
Import the combineReducers helper function at the beginning of the src/reducers.js file:
import { combineReducers } from 'redux'
Then call combineReducers with our reducer functions at the end of the src/reducers.js file. It returns an appReducer function:
const appReducer = combineReducers({
posts: postsReducer,
filter: filterReducer,
})
We export default the main reducer, by adding the following line at the end of the file:
export default appReducer
Now, you can import the reducer in the src/index.js file, as follows:
import appReducer from './reducers'