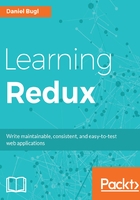
Creating the store
We will now cover how to use Redux to create a store from our appReducer, how to listen to changes in the store, and how to dispatch actions to the store:
First of all, we will need to import the createStore function from the Redux library. Add the following code at the beginning of the src/index.js file:
import { createStore } from 'redux'
The createStore function takes the following arguments:
- A reducer function (the main/root reducer, in our case, appReducer) as the first argument.
- An initial state as the second argument--if no initial state is specified, the store will be initialized by passing undefined to the reducer, and thus, using the default value returned by it.
The createStore function returns the Redux store, which provides some functions to access the state (store.getState()), listen to state changes (store.subscribe(listener)), and dispatch actions (store.dispatch(action)):
let store = createStore(reducer, initialState)
We use the createStore function to create the store from our previously created appReducer. Instead of manually calling the reducer, we replace the rest of the code in src/index.js with the following:
let store = createStore(appReducer)
That's basically all there is to it; now we can use the store. Let's try logging the initial state:
console.log(store.getState())
The initial state looks exactly as we expected—it contains the default values specified in the reducers:
{
"posts": [],
"filter": "all"
}