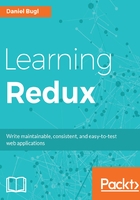
Handling the user input
To add some interactivity (and show how to dispatch actions from the user interface), we will implement a feature that adds an exclamation mark to a post when it is clicked.
We just need to add a click event listener to each post. In this event listener, we store.dispatch() an editPost action that adds a ! to the post text. We can do this in our render() function:
const render = () => {
root.innerHTML = ''
const { posts } = store.getState()
posts.forEach((post, index) => {
const item = document.createElement('li')
item.addEventListener('click', () =>
store.dispatch(editPost(index, post.text + '!'))
)
const text = document.createTextNode(post.user + ' - ' + post.text)
item.appendChild(text)
root.appendChild(item)
})
}
Note how we use the index (which forEach passes as a second argument to the callback function) and the current post text (post.text) to create the editPost action.
Try clicking on a post, and see how the exclamation mark gets added:

The render() function we created is actually already very similar to how React works. Given the current state of the application, we simply describe what needs to be rendered. When the state changes, render() gets called again, and the application gets re-rendered. However, React does this in a smart way—it only re-renders the parts of the interface that changed. It also offers a better way to describe your user interface using JSX syntax. We will learn more about React in the next chapter.