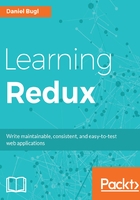
Creating a simple React element
Just like other JavaScript values, you can store React elements in a variable/constant:
const greeting = <h1>hello world!</h1>
If you have JSX code that spans across multiple lines, you can wrap it in ( and ) brackets, as follows:
const greeting = ( <h1> hello world! </h1> )
There is something else that makes JSX special—you can embed JavaScript expressions in JSX by wrapping them in curly brackets { }. That way, you can display variables/constants, evaluate functions, show their result, and so on. Let's display a name constant instead of world:
const name = 'dan' const greeting = ( <h1> hello {name}! </h1> )
We could also show the name in uppercase, by calling .toUpperCase() on the constant:
const name = 'dan' const greeting = ( <h1> hello {name.toUpperCase()}! </h1> )
In JSX, all JavaScript expressions are valid when written in curly brackets { }. They will be evaluated, and the result will be inserted in place of the expression. In our example, the following HTML code will be rendered:
<h1>hello DAN!</h1>