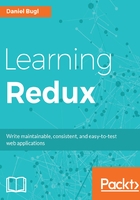
Creating a static class component
Now, we can turn this functional component into a class by performing the following steps:
We start with the definition:
class Timer extends React.Component {
Classes in ES2015 JavaScript work similar to classes in Java. They contain functions (methods) and variables/constants. Although you have to be careful, as there are some differences when it comes to inheritance. However, you should not have to deal with these differences, because when using React, you should favor composition (wrapping components in other components) over inheritance (extending components from other components).
Next, we need to define a render method, which will be called to render the component. It returns React elements, which means that we can use JSX here:
render () {
With class components, properties are stored in this.props and not passed to the render method. We can also use de-structuring here to pull out the properties we need:
const { seconds } = this.props
Now, we return the to-be-rendered content via JSX:
return <h1>You spent {seconds} seconds on this page!</h1> } }