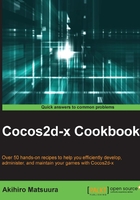
Getting the sprite's position and size
There is a certain size and position of the sprite. In this recipe, we explain how to view the size and position of the sprite.
How to do it...
To get the sprite position, use the following code:
Vec2 point = sprite->getPosition(); float x = point.x; float y = point.y;
To get the sprite size, use the following code:
Size size = sprite->getContentSize(); float width = size.width; float height = size.height;
How it works...
By default, the sprite position is (0,0
). You can change the sprite position using the setPosition
method and get it using the getPosition
method. You can get the sprite size using the getContentSize
method. However, you cannot change the sprite size by the setContentSize
method. The contentsize
is a constant value. If you want to change the sprite size, you have to change the scale of the sprite. You will learn about the scale in the next recipe.
There's more...
Anchor point is a point that you set as a way to specify what part of the sprite will be used when setting its position. The anchor point uses a bottom-left coordinate system. By default, the anchor point of all Node objects is (0.5, 0.5)
. This means that the default anchor point is the center.
To get the anchor point at the center of the sprite, we use the following code:
sprite->setAnchorPoint(Vec2(0.5, 0.5));
To get the anchor point at the bottom-left of the sprite, we use the following code:
sprite->setAnchorPoint(Vec2(0.0, 0.0));
To get the anchor point at the top-left of the sprite, we use the following code:
sprite->setAnchorPoint(Vec2(1.0, 0.0));
To get the anchor point at the bottom-right of the sprite, we use the following code:
sprite->setAnchorPoint(Vec2(0.0, 1.0));
To get the anchor point at the top-right of the sprite, we use the following code:
sprite->setAnchorPoint(Vec2(1.0, 1.0));
The following image shows the various positions of the anchor point:

To get the sprite rectangle, use the following code:
Rect rect = sprite->getBoundingBox(); Size size = rect.size; Vec2 point = rect.origin;
Rect
is the sprite rectangle that has properties such as Size
and Vec2
. If the scale is not equal to one, then Size
in Rect
will not be equal to the size
, using getContentSize
method. Size
of getContentSize
is the original image size. On the other side, Size
in Rect
using getBoundingBox
is the size of appearance. For example, when you set the sprite to half scale, the Size
in Rect
using getBoundingBox
is half the size, and the Size
using getContentSize
is the original size. The position and size of a sprite is a very important point when you need to specify the sprites on the screen.
See also
- The Detecting collisions recipe, where you can detect collision using
rect
.