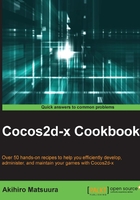
Creating actions
Cocos2d-x has a lot of actions, for example, move, jump, rotate, and so on. We often use these actions in our games. This is similar to an animation, when the characters in a game start their action, the game will come alive. In this recipe you will learn how to use a lot of actions.
How to do it...
Actions are very important effects in a game. Cocos2d-x allows you to use various actions.
To move a sprite by a specified point over two seconds, you can use the following command:
auto move = MoveBy::create(2.0f, Vec2(100, 100)); sprite->runAction(move);
To move a sprite to a specified point over two seconds, you can use the following command:
auto move = MoveTo::create(2.0f, Vec2(100, 100)); sprite->runAction(move);
To uniformly scale a sprite by 3x over two seconds, use the following command:
auto scale = ScaleBy::create(2.0f, 3.0f); sprite->runAction(scale);
To scale the X
axis by 5x, and Y
axis by 3x over two seconds, use the following command:
auto scale = ScaleBy::create(2.0f, 5.0f, 3.0f); sprite->runAction(scale);
To uniformly scale a sprite to 3x over two seconds, use the following command:
auto scale = ScaleTo::create(2.0f, 3.0f); sprite->runAction(scale);
To scale X
axis to 5x, and Y
axis to 3x over two seconds, use the following command:
auto scale = ScaleTo::create(2.0f, 5.0f, 3.0f); sprite->runAction(scale);
To make a sprite jump by a specified point three times over two seconds, use the following command:
auto jump = JumpBy::create(2.0f, Vec2(20, 20), 20.0f, 3); sprite->runAction(jump);
To make a sprite jump to a specified point three times over two seconds, use the following command:
auto jump = JumpTo::create(2.0f, Vec2(20, 20), 20.0f, 3); sprite->runAction(jump);
To rotate a sprite clockwise by 40 degrees over two seconds, use the following command:
auto rotate = RotateBy::create(2.0f, 40.0f); sprite->runAction(rotate);
To rotate a sprite counterclockwise by 40 degrees over two seconds, use the following command:
auto rotate = RotateTo::create(2.0f, -40.0f); sprite->runAction(rotate);
To make a sprite blink five times in two seconds, use the following command:
auto blink = RotateTo::create(2.0f, -40.0f); sprite->runAction(blink);
To fade in a sprite in two seconds, use the following command:
auto fadein = FadeIn::create(2.0f); sprite->runAction(fadein);
To fade out a sprite in two seconds, use the following command:
auto fadeout = FadeOut::create(2.0f); sprite->runAction(fadeout);
The following code skews a sprite's X
axis by 45 degrees and Y
axis by 30 degrees over two seconds:
auto skew = SkewBy::create(2.0f, 45.0f, 30.0f); sprite->runAction(skew);
The following code skews a sprite's X
axis to 45 degrees and Y
axis to 30 degrees over two seconds.
auto skew = SkewTo::create(2.0f, 45.0f, 30.0f); sprite->runAction(skew);
How it works...
Action
objects make a sprite perform a change to its properties. MoveTo
, MoveBy
, ScaleTo
, ScaleBy
and others, are Action
objects. You can move a sprite from one position to another position using MoveTo
or MoveBy
.
You will notice that each Action
has a By
and To
suffix. That's why they have different behaviors. The method with the By
suffix is relative to the current state of sprites. The method with the To
suffix is absolute to the current state of sprites. You know that all actions in Cocos2d-x have By
and To
suffix, and all actions have the same rule as its suffix.
There's more...
When you want to execute a sprite action, you make an action and execute the runAction
method by passing in the action
instance. If you want to stop the action while sprites are running actions, execute the stopAllActions
method or stopAction
by passing in the action
instance that you got as the return value of the runAction
method.
auto moveTo = MoveTo::create(2.0f, Vec2(100, 100)); auto action = sprite->runAction(moveTo); sprite->stopAction(action);
If you run stopAllActions
, all of the actions that sprite is running will be stopped. If you run stopAction
by passing the action
instance, that specific action will be stopped.