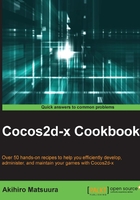
Calling functions with actions
You may want to call a function by triggering some actions. For example, you are controlling the sequence action, jump, and move, and you want to use a sound for the jumping action. In this case, you can call a function by triggering this jump action. In this recipe, you will learn how to call a function with actions.
How to do it...
Cocos2d-x has the CallFunc
object that allows you to create a function and pass it to be run in your Sequence
. This allows you to add your own functionality to your Sequence
action.
auto move = MoveBy::create(2.0f, Vec2(100, 0)); auto rotate = RotateBy::create(2.0f, 360.0f); auto func = CallFunc::create([](){ CCLOG("finished actions"); }); auto action = Sequence::create(move, rotate, func, nullptr); sprite->runAction(action);
The preceding command will execute the following actions sequentially:
- Move a sprite 100px to the right over two seconds
- Rotate a sprite clockwise by 360 degrees over two seconds
- Execute CCLOG
How it works...
The CallFunc
action is usually used as a callback function. For example, if you want to perform a different process after finishing the move
action. Using CallFunc
, you can call the method at any time. You can use a lambda expression as a callback function.
If you get a callback with parameters, its code is the following:
auto func = CallFuncN::create([](Ref* sender){ CCLOG("callback"); Sprite* sprite = dynamic_cast<Sprite*>(sender); });
The instance of this parameter is the sprite that is running the action. You can get the sprite instance by casting to Sprite
class.
Then, you can also specify a callback method. CallFunc
has CC_CALLBACK_0
macro as an argument. CC_CALLBACK_0
is a macro for calling a method without parameters. If you want to call a method with one parameter, you need to use the CallFuncN
action and CC_CALLBACK_1
macro. CC_CALLBACK_1
is a macro for calling a method with one argument. A parameter of a method that is called by CallFuncN
is the Ref
class.
You can call a method using the following code:
bool HelloWorld::init() { … auto func = CallFunc::create(CC_CALLBACK_0(HelloWorld::finishedAction, this)); … } void HelloWorld::finishedAction() { CCLOG("finished action"); }
To call a method with an argument, you can use the following code:
bool HelloWorld::init() { … auto func = CallFuncN::create(CC_CALLBACK_1(HelloWorld::callback, this)); … } void HelloWorld::callback(Ref* sender) { CCLOG("callback"); }
There's more...
To combine the CallFuncN
and the Reverse
action, use the following code:
auto move = MoveBy::create(2.0f, Vec2(100, 0)); auto rotate = RotateBy::create(2.0f, 360.0f); auto func = CallFuncN::create([=](Ref* sender){ Sprite* sprite = dynamic_cast<Sprite*>(sender); sprite->runAction(move->reverse()); }); auto action = Sequence::create(move, rotate, func, nullptr); sprite->runAction(action);
The preceding command will execute the following actions sequentially:
- Move a sprite 100px to the right over two seconds
- Rotate a sprite clockwise by 360 degree over two seconds
- Move a sprite 100px to the left over two seconds