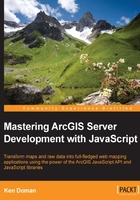
Tasks
As I mentioned in the previous chapter, some application items require resource-intense processes that are best left for the server. The ArcGIS API calls these actions tasks. There are a large variety of tasks. Some require the server to compute and/or return data, which is the case for the geometry service and the QueryTask
. Others use the server to generate custom documents, like the Print Task. Still, others also do all this, and also require custom extensions not initially a part of ArcGIS Server. Let's take a look at what makes a good task.
Tasks, parameters, and results
As we discussed in the previous chapter, a task is made up of three parts, the task constructor, the task parameters, and the task results. Typically, only the task constructor and the task parameters need to be loaded using AMD. The task results are automatically produced when the results return from the server. The format of the results is shown in the API documentation to show you how to access what you need when you successfully receive results. The common order of events with tasks is as follows:
- The task and task parameters are constructed.
- The task parameters are filled in with the required information
- The task executes a server call using the task parameters.
- When the browser receives the results from the server, something is done with the results either through a
callback
function or a deferred value.
It is worth noting that when tasks are executed, they return one of Dojo's Deferred objects. These deferred results can be passed to another function to be run asynchronously when the browser receives the results.
Common tasks
There are many tasks commonly available on any installation of ArcGIS Server. Many rely on a published map service or geoprocessing service to act on. Some, like the GeometryService
, are built into ArcGIS Server. Let's take a look at some of the common tasks available through the API.
GeometryService
provides multiple functions for manipulating geometry data. Typically, it is published as part of ArcGIS Server. Let's take a look at the Geometry Service functions available.
AreasAndLengths
: Finds areas and perimeters of polygon geometriesAutoComplete
: Helps create polygons adjacent to other polygons by filling in gaps between themBuffer
: Creates a polygon whose edges are a set distance or distances from the source geometryConvexHull
: Creates the smallest polygon shape necessary to contain all the input geometriesCut
: Splits a polyline or a polygon along a secondary polylineDifference
: Takes a list of geometries, and a secondary geometry (probably a polygon), and returns the first list any overlap against the secondary geometry is cut outDistance
: Finds the distance between geometriesGeneralize
: Draws a similar looking shape with much fewer pointsIntersect
: Given a list of geometries and another geometry, returns the geometries defined by where the first list intersected with the secondLabel Points
: Finds a point within a polygon which would be the best position to place a labelLengths
: For lines and polylines, finds the planar or geodesic distance from beginning to endOffset
: Given a geometry and a distance, will create a geometry the defined distance to the right of the original geometry if the distance is positive, or to the left of the geometry if negativeProject
: Based on a geometry and a new spatial reference, it returns the original geometry in the new spatial referenceRelation
: Based on two sets of geometries and a specified relationship parameter, returns a list of pairings showing how items in the two lists are related (for example: how many from list2 are inside list1)Reshape
: Given a line or a polygon, and a secondary line, it changes the shape of the first geometry based on the second one, adding to the original figure, or tossing some of it awaySimplify
: Given a complicated polygon or line whose edges crosses itself, it returns simpler polygons where the crossed parts are undoneTrimExtend
: Given a list of polylines, and a polyline to trim or extend to, it cuts lines in the list where they cross over the second polyline, and extend other polylines where they don't quite reach the secondary polylineUnion
: Given a list of polygons, creates a unified geometry where all the polygons are dissolved along edges that overlap, creating one solid polygon
The QueryTask
provides a way to collect both shape and attribute data from features in a map service. You can request data on individual layers in a map service, defining your search area by geometry and/or limiting your results to attribute queries. The QueryTask
is constructed with a URL parameter pointing to a single layer in a map service. The number of results returned from a successful query is limited by ArcGIS Server, typically to either 1,000 or 2,000 results.
Because results for a QueryTask
can be limited by ArcGIS Server, the QueryTask
object has been extended with multiple methods to collect data on its search results. Here are some of the methods available through the QueryTask
:
execute()
: Accepts theQueryTask
parameters, and requests features based on those parameters.executeForCount()
: Accepts the same parameters, and returns the number of results in the map service layer that match the query.executeForExtent()
: It is based on the parameters passed, returns the geographic extent that contains all the search results. Note that this is only valid on hosted feature services on www.arcgis.com.executeForIds()
: With the parameters passed, the results return a list ofObjectIds
for the features that match the search. Unlike the execute command, where the number of results are limited by ArcGIS Server, theexecuteForIds()
method returns all theObjectIds
that match the search. That means, if there are 30,000 results that match the search, this method will return 30,000ObjectIds
, instead of the server limit of 1,000.
The QueryTask
parameters can be created using the Query
module (esri/tasks/query
). If you have experience with SQL, you'll see similarities between the Query
parameters and SQL Select
statements, while there are some Query
parameters that are more specific to ArcGIS Server. A few of the common ones are as follows:
where
(string): A legal where clause for a query.geometry
(esri/geometry): A shape to spatial select against.returnGeometry
(Boolean): Whether you want the geometry of the search result features. Setting this to false returns just attribute data.outFields
(array of strings): A list of fields to retrieve the search results. Assigning ["*
"] to theoutFields
parameter returns all fields, which is equivalent to the SQL statementSELECT * from
.outSpatialReference
(esri/SpatialReference
): The spatial reference you want the geometry to be drawn in. Typically, the map's spatial reference is assigned to this value.orderByFields
(array of strings): A list of field values to sort the results by. AddDESC
to the string if you want values returned in descending order.
Results are returned from QueryTask
in a form called FeatureSet
(esri/tasks/FeatureSet
). FeatureSet
contains data about the results returned, including the geometry type of the results, the spatial reference, field aliases for the results, and a list of feature graphics that match the search results. The featureSet
even returns whether or not the number of results that match the query exceeded the number that could be delivered by ArcGIS Server. The array of features within the features property of the featureSet
can symbolized and added straight to a map's graphics layer to be seen and clicked.
The IdentifyTask
provides a shortcut way to retrieve data from a map service for a popup. Unlike the QueryTask
, which queries only one layer in the map service, the IdentifyTask
can search through all layers in the map service. While the QueryTask
has numerous methods to retrieve data on a search, the IdentifyTask
is more simplified, with a single execute()
command to retrieve server results.
The parameters passed to an IdentifyTask
are referred to as IdentifyParameters
. Because IdentifyTasks
can search multiple layers, the IdentifyParameters
focus on searching by geometry. To search by a where
clause, a layerDefinition
parameter must also be added to the parameters.
The results of an IdentifyTask
, known as the IdentifyResults
, differ somewhat from the FeatureSet
. First, IdentifyResults
are returned as an array of objects that contain a feature parameter that contains the result graphic, while the FeatureSet
is a single object with an array of graphics in its features parameter. Secondly, IdentifyResults
do not contain a list of field names and aliases like the FeatureSet
, because the feature attributes of the IdentifyResult
are name/value pairs where the name key is the alias. Finally, the IdentifyResults
return the layer id and name of the layer they were retrieved from. These attributes makes the IdentifyTask
favourable to quickly populate a map's popup on a click.
The ArcGIS API offers another tool for finding features on a map called a FindTask
. The FindTask
accepts a text string, and searches for features in the map service that contain that value. On the database side, the FindTask
queries through each layer in the map service, searching permitted fields for a text string with wildcards before and after it. If searching for the string "Doe", the FindTask
would return a feature with the name value of "John Doe", "Doe Trail", or "Fiddledee
doe Studios", but would not return a feature containing "Do exit" because of the space.
A Locator provides either an approximate location for an address based on street addressing data, known as geocoding. The locator can also perform reverse geocoding, which provides an approximate address for a point on the map based on that same street data. ESRI provides geocoding services on a national and world level, but if you have more up-to-date street information, you can publish your own geocoding service on ArcGIS Server.
For address location, the locator accepts a parameter object containing either a single line input, or an object containing a Street, City, State, and Zone (zip code) parameter. The callback
function that accepts the Locator.execute()
results should accept a list of objects called AddressCandidates
. These AddressCandidates
include the possibly matching street address, the point location of the address, and a score from 0 to 100, where 100 is a perfect match. If no results return there were no matches for the address provided.
Tasks requiring server extensions
While ArcGIS Server packs a lot of functionality, ESRI also provides server extensions, or optional software that performs specific tasks through ArcGIS Server. This software can do a number of unique tasks, from providing driving directions to sifting through tweets in your area. You will need to know if your server has one or more of these extensions before trying to use them. We'll take a look at one of them, the Routing Task.
Routing Tasks can provide driving directions, calculate the most effective routes, and redirect users around known roadblocks. The Routing Tasks require the Network Analyst extension for ArcGIS Server. For Routing Parameters, you can add a FeatureSet
into the stop parameter, and fill it with graphics defining the various stops our vehicle has to make. You can also supply barriers in the same way, which block certain roadways. When the Routing Task is executed, it returns a best route based on the street data provided to the service.