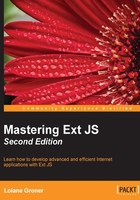
Creating the application with Sencha Cmd
Let's get started and get our hands on the code. The first thing we are going to do is create the application using the MVC structure. Sencha Command (referred to as Sencha Cmd from now on) provides the capability of creating the application structure automatically for us. Utilizing Sencha Cmd is helpful not only because it creates the structure according to the MVC architecture for our application, but also because it provides all the files we need later on when going live with the software and to customize its theme—we'll learn more about this in a later chapter.
A quick word about MVC
MVC stands for Model-View-Controller. It is a software architecture pattern that separates the representation of the information from the user's interaction with it. The Model represents the application data, the View represents the output of the representation of the data (form, grid, chart), and the Controller mediates the input, converting it into commands for the Model or View.
Ext JS supports MVC, which is a Model-View-Controller pattern (as one of the architecture options, it also provides a Model-View-View-Model (MVVM), which we will discuss later). The Model is a representation of the data we want to manipulate in our application, a representation of a table from the database. The View is all the components and screens we create to manage the information of a Model. As Ext JS is event-driven, all the View instances fire events when the user interacts with them, the Controller can be configured to listen to events raised from the View, and the developer can implement custom handlers to respond to these events. The Controller can also redirect the command to the Model (or Store) or the View. And the Store in Ext JS is very similar to the Data Access Object (DAO) pattern used on server-side technologies (with more capabilities such as sorting and filtering, introduced in Chapter 1, Sencha Ext JS Overview).
For a quick example, let's say we have WidgetA, which is a GridPanel that displays all the records from a table. This table is represented by ModelA. StoreA is responsible for retrieving the information (collection of ModelA from the server). When the user clicks on a record from WidgetA, a window will be opened (called WidgetB) displaying information from a table (represented by ModelB). And of course, StoreB will be responsible for retrieving the collection of ModelB from server. In this case, we will have ControllerA to capture the itemclick
event from WidgetA, do all the required logic to display WidgetB, and load all the ModelB information. If we try to put this into a quick reference diagram, it would be something like this:

Creating the application
We are going to create the application inside the htdocs
folder of our Xampp directory. Our application will be named masteringextjs
.
Before we start, let's take a look how the htdocs
folder looks:

We still have the original Xampp files in there, and the Ext JS 5 SDK folder, along with the Ext JS 5 documentation folder.
The next step is to use Sencha Cmd to create the application for us. To do so, we need to open the terminal application that comes with the operating system we 're using. For Linux and Mac OS users, this would be the terminal application. For Windows users, it's the Command Prompt application.
Here are the steps that we are going to execute:
- First, we need to change the current directory to the Ext JS directory (
htdocs/ext-5.0.0
in this case). - Then, we will use the following command:
sencha generate app Packt ../masteringextjs
The sencha generate app
command will create the masteringextjs
directory inside the htdocs
folder with the necessary file structure required by the MVC architecture as used by ExtJS. Packt
is the name of the namespace of our application, which means that every class we create is going to start with Packt
, for example, Packt.model.Actor
, Pack.view.Login
, and so on. And the last argument passed to the command is the directory where the application will be created. In this case, it is inside a folder named masteringextjs
, which is located inside the htdocs
folder.
Note
Namespaces are used for scoping variables and classes so that they are not global, defining deeply their nested structures. Sencha has a good article about namespaces at http://goo.gl/2iLxcn.
After the command finishes its execution, we will have something like what is shown in the following screenshot:

Note
The source code presented in this book was written with Ext JS 5.0 (so you will see the screenshots with version 5.0) and upgraded to 5.1 once it was released. So once you download its source code, it will be compatible with version 5.1. Upgrading the source code from 5.0 to 5.1 does not have any impact.
But why do we need to create a project structure like this one? The following is the structure used by Ext JS applications:

Note
For more information about the Sencha generate app
command, please consult http://docs.sencha.com/cmd/5.x/extjs/cmd_app.html.
Let's see what each folder does.
First we have the app
folder. This is where we will create all the code for our application. Inside the app
folder, we can find the following folders as well: controller
, model
, store
, and view
. We can also find the Application.js
file. Let's have a detailed look at them. In the model
folder, we will create all files that represent a Model, which is an Ext JS class that represents a set of fields, which means that an object that our application manages (actor, country, film, and so on). It is similar to a class on the server side with only the attributes of the class plus the getter and setter methods used to represent a table from the database.
On the store
folder, we will create all the Store
classes, which are caches of collections of Model instances. The store
folder also has capabilities similar to DAO—classes used on server-side languages to perform CRUD operations on the database. As Ext JS does not communicate directly with databases, the Store
classes are used to communicate with the server side or a local storage—used with a Proxy. Proxies are used by Store or Model instances to handle the loading and saving of Model data, and it is the place where we are going to configure how we want to communicate with the server (using Ajax and using JSON or XML, formatting the data so that the client and the backend understand each other).
On the view
folder, we will create all view
classes, also known as the User Interface Components (UI Components), such as grids, trees, menus, forms, windows, and so on.
And finally, in the controller
folder, we can create classes that will handle the events fired by the components (events fired because of the life cycle of the component or because of some interaction of the user with a component). We always need to remember that Ext JS is event-driven, and on the Controller
classes, we will control these events and update any Model, View, or Store (if required). Some examples of events fired would be a click or mouseover of a button, an itemclick
event of a row of a grid, and so on.
The MVC app
folder structure has been created. Now, we will copy the Ext JS SDK (extjs
folder) into the masteringExtjs
folder, and we will also create a file named app.js
. We will edit this file later in this chapter.
We also have the Application.js
file. This is the entry point of our application. We'll come back to this later on.
The masteringextjs
directory contains a few more files:
app.js
: This file inherits the code from theApplication.js
file. It is the point of entry of the application. This is the file that is called by Ext JS to initialize the application. We should avoid changing this fileapp.json
: this is a configuration file for the application. In this file, we can add extra CSS and JS files, which should be loaded with the application, such as charts and locale-specific configurations. We will make some changes in this file throughout the book.bootstrap.css
,bootstrap.json
, andbootstrap.js
: Created by Sencha Cmd, these files should not be edited. The CSS file contains the import of the theme used by the application (which is the so-called Neptune theme by default). After we make a build, the content of these files is updated with CSS definitions, and the JavaScript files will contain dependencies that need to be loaded prior to execution, customxtypes
, and other class system features.build.xml
: Sencha Cmd uses Apache Ant (http://ant.apache.org/), which is a Java tool used to build Java projects. Ant uses a configuration file namedbuild.xml
, which contains all the required configurations and commands to build a project. Sencha Cmd uses Ant as the engine to build an Ext JS application on the background (while we simply need to use a command). This is the reason why we need to have the Java SDK installed to use some of the Sencha Cmd features.index.html
: This is the index file of our project. This is the file that will be rendered by the browser when we execute our application. Inside this file, we will find the import of thebootstrap.js
file. We should avoid making changes in this file as well because when we build the application, Sencha Cmd will generate a newindex.html
file in thebuild
folder, discarding all the changes we might have made to theindex.html
file. If we need to include a JS or CSS file, we should define this within theapp.json
file.ext
: Inside this folder, we can find all the Ext JS framework files (ext-all
,ext-all-debug
, andext-dev
), its source code, and also thepackages
folder, containing the locale definitions for our application and theme-related packages, among others.overrides
: When we create the application, this folder is empty. We can add any class overrides and customizations we will need for our project.packages
: Inside this folder, we can create our own packages. A package can be a new theme, for example. Its concept is similar to gems in Ruby or custom APIs in Java and .NET (for example, to use Apache Ant in a Java project, we need to include the Apache Antjar
file).resources
: Inside this folder, we will place all the images of our application. We can also place other CSS files and font files.sass
: Inside this folder, we can find some Sass files used to create themes. Any custom CSS for our application will be created inside this folder.
Let's gain firsthand knowledge now! We will now explore some of the concepts described previously during the development of our application to understand them better.
Sencha Cmd has another command that is very useful while developing Ext JS applications. It is the watch
command. We will use this command all the time while developing the application in this book.
Let's execute this command and see what it does. First, we need to change the directory to the masteringextjs
folder (which is our application folder generated with Sencha Cmd). Then, we can execute the sencha app watch
command. The following screenshot exemplifies the output of this command:

This command looks for any changes made inside the Ext JS application folder. If we create a new file or change any file, this command will know that a change has been made and will make an application build.
It is also going to start a web server at http://locahost:1841
, where we can access our application as demonstrated in the following screenshot:

So this is how an application created with Sencha Cmd looks. Sencha Cmd also creates some files inside the app
folder that we can change according to our needs. We will go through the files in the next chapter.
We can also execute the application from the Xampp localhost
URL by accessing http://localhost/masteringextjs
, as follows:

Tip
The output from http://localhost/masteringextjs
is exactly the same as accessing http://locahost:1841
. You can use any of them. But be aware that http://locahost:1841
will be alive only when we use the sencha app watch
command. As we will use PHP and apply some other configurations, we will use http://localhost/masteringextjs
throughout the book.
We know that Sencha Cmd has created some files for us, but we do not want that screen to be the first one the user will see. We want the user to see the login screen and then go the main screen.
To make it happen, we need to make some changes inside the app.js
file. If we open the file, we will see the following content:
Ext.application({
name: 'Packt',
extend: 'Packt.Application',
autoCreateViewport: 'Packt.view.main.Main'
});
We are going to change the preceding code that was highlighted to the following code:
autoCreateViewport: false
This is the only change we will make in this file, because we should avoid changing it.
What this line does is create the Packt.view.main.Main
component automatically after the application is initialized, but we do not want that happening. We want to display the splash screen first and then the login screen. That is why we are asking the application not to auto-render the viewport.
Note that the terminal where we have the sencha app watch
command running will output a few lines, which means the application will be refreshed and the development build updated. This will happen every time we make a change and save it.
If we refresh the browser, we should see an empty page. This means we can start the development of our DVD Rental Store application.
If we open the app/Application.js
file, this is how it looks:
Ext.define('Packt.Application', { // #1 extend: 'Ext.app.Application', name: 'Packt', // #2 views: [ // #3 ], controllers: [ // #4 'Root' ], stores: [ // #5 ], launch: function () { // #6 // TODO - Launch the application } });
The app.js
file inherits all the behavior of the application from the Application.js
file. This file is used as the entry point of the app.
On the first line of the preceding code, we have the Ext.application
declaration (#1
). This means that our application will have a single page (http://en.wikipedia.org/wiki/Single-page_application), and the parent container of the app will be the viewport. The viewport is a specialized container representing the viewable application area that is rendered inside the <body>
tag of the HTML page (<body></body>
).
Inside the Ext.application
, we can also declare views
(#3
), controllers
(#4
), and stores
(#5
) used by the application. We will add this information in this file as we create new classes for our project.
We need to declare the name
attribute of the application, which will be the namespace (#2
) of the application. In this case, Sencha Cmd used the namespace we used in the sencha generate app
command.
We can also create a launch
function inside Ext.application
(#6
). This function will be called after all the application's controllers are initialized, and this means that the application is completely loaded. So this function is a good place to instantiate our main view, which in our case will be the login screen.
Tip
Do we need to use Ext.onReady
when using Ext.application
?
The answer is no. We only need to use one of the options. According to the Ext JS API documentation, Ext.application
loads Ext.app.Application class and starts it up with given configuration after the page is ready and Ext.onReady
adds a new listener to be executed when all required scripts are fully loaded.
If we take a look at the source code for Ext.application
, we have:
Ext.application = function(config) { Ext.require('Ext.app.Application'); Ext.onReady(function() { new Ext.app.Application(config); }); };
This means that Ext.application
is already calling Ext.onReady
, so we do not need to do it twice.
Hence, use Ext.onReady
when you have a few components to be displayed, which are not in the MVC architecture (similar to the jQuery $(document).ready()
function), and use Ext.application
when you are developing an Ext JS MVC application.
The following diagram exemplifies all the high-level steps performed during the Ext JS application startup. Once the steps are executed, the application is completely loaded:

Now that we know how an Ext JS application is initialized, we can start building our app.