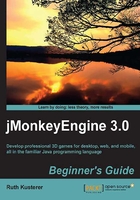
Time for action – starting the application
In the main()
method of the class, you need to create a new instance of the application and call its start()
method. In this case, the main class is called Main
, but you can also name it MyGame or whatever you like:
public static void main(String[] args) { Main app = new Main(); app.start(); }
When you run this code, the application window opens and the jMonkeyEngine starts rendering the scene.
What just happened?
Every jMonkeyEngine application provides the following methods for starting and stopping the game:

Tip
When a user presses the Alt + Tab shortcut or clicks outside the game window, the running application loses focus. You can set app.setPauseOnLostFocus
as true,
to specify that your game should pause whenever the window loses focus. For real-time or online games, set app.setPauseOnLostFocus as false
instead, to keep the game running.
Let's sum up what we just learned. When you write a jMonkeyEngine game, you have to:
- Extend
com.jme3.app.SimpleApplication
- Implement abstract methods, such as
simpleInitApp()
,simpleUpdate(float tpf)
, andsimpleRender(RenderManager rm)
(described later in the chapter) - Call
app.start()
on your instance ofSimpleApplication
The jMonkeyEngine runs the application even if you leave the three method bodies empty. If you run a SimpleApplication
class without initializing any scene, the screen shows up empty.