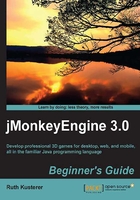
Time for action – mapping meets listeners
To activate the mappings, you have to register them to an InputListener
object. The jMonkeyEngine offers several InputListener
objects in the com.jme3.input.controls.*
package. Let's create instances of the two most common InputListener
objects and compare what they do.
- On class level, below the closing curly braces of the
simpleInitApp()
method, add the following code snippet:private ActionListener actionListener = new ActionListener() { public void onAction(String name, boolean isPressed, float tpf) { System.out.println("You triggered: "+name); } }; private AnalogListener analogListener = new AnalogListener() { public void onAnalog(String name, float intensity, float tpf) { System.out.println("You triggered: "+name); } };
Tip
When you paste code in the jMonkeyEngine SDK's editor, unknown symbols are underlined in red. Whenever you see yellow warnings next to lines, click on the lightbulb icon and execute the hint to resolve the problem, in this case, Add import for... Alternatively, you can also press the Ctrl + Shift + I shortcut keys to fix all import statements in one go.
- Register each mapping to one of the
InputListener
objects. Paste the following two lines of code below theaddMapping()
code lines in thesimpleInitApp()
method:inputManager.addListener(actionListener, new String[]{MAPPING_COLOR}); inputManager.addListener(analogListener, new String[]{MAPPING_ROTATE});
If you run the code sample now, and then click, or press the C key or Space bar, you should see some output in the console.
What just happened?
jMonkeyEngine comes with three preconfigured InputListener
objects: ActionListener
, AnalogListener
, and TouchListener
. Each responds differently to com.jme3.input.event.InputEvents
.
ActionListener
is optimized for discrete either/orInputEvents
. Use it if you want to detect whether a key is either pressed or released, or the mouse button is either up or down, and so on.AnalogListener
is optimized for continuousInputEvents
with an intensity. This includes mouse or joystick movements, (for example, when looking around with the camera), or long-lasting key presses (for example, when walking by pressing the W, A, S, and D keys), or long mouse clicks (for example, when the player shoots an automatic weapon).TouchListener
is optimized forInputEvents
on touchscreen devices. It supports events, such as tap, double-tap, long pressed tap, fling, and two-finger gestures.
In the previous example, you registered MAPPING_COLOR
to the actionListener
object, because toggling a color is a discrete either/or decision. You have registered the MAPPING_ROTATE
mapping to the analogListener
object, because rotation is a continuous action. Make this decision for every mapping that you register. It's common to use both InputListener
objects in one game, and to register all analog actions to one and all discrete actions to the other listener.
Tip
Note that the second argument of the addListener()
method is a String array. This means that you can add several comma-separated mappings to one InputListener
object in one go. It is also perfectly fine to call the inputManager.addListener()
method more than once in the same application, and to add a few mappings per line. This keeps the code more readable. Adding more mappings to an InputListener
object does not overwrite existing mappings.