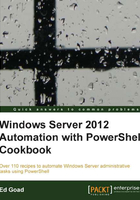
Creating AD users
When working in a test or lab environment, it is useful to have a number of test accounts to use. These accounts can have different access permissions and simulate different types of users doing specific tasks. These AD users are normally made up of simple accounts with a common password.
Additionally, when setting up a new production environment, it may be necessary to populate users into AD. These usernames and e-mail addresses are predefined and the passwords must be unique.
In this recipe we will use a PowerShell script to create both types of users.
Getting ready
To use this recipe properly, you need an AD environment with credentials capable of creating user accounts. Additionally, if you want to create specific users, you will need a CSV file with headers of LastName,FirstName as shown in the following screenshot that contains the users to create:

How to do it...
Carry out the following steps to create AD users:
- To create a single Active Directory user account, use the following command:
New-ADUser -Name JSmith
- To create multiple Active Directory user accounts, we use the following functions:
Function Create-Users{ param($fileName, $emailDomain, $userPass, $numAccounts=10) if($fileName -eq $null ){ [array]$users = $null for($i=0; $i -lt $numAccounts; $i++){ $users += [PSCustomObject]@{ FirstName = 'Random' LastName = 'User' + $i } } } else { $users = Import-Csv -Path $fileName } ForEach($user in $users) { $password = '' if($userPass) { $password = $userPass } else { $password = Get-RandomPass } Create-User -firstName $user.FirstName ` -lastName $user.LastName -emailDomain $emailDomain ` -password $password } } Function Create-User { param($firstName, $lastName, $emailDomain, $password) $accountName = '{0}.{1}' -f $firstName, $lastName $emailAddr = '{0}@{1}' -f $accountName, $emailDomain $securePass = ConvertTo-SecureString $password -AsPlainText -Force New-ADUser -Name $accountName -AccountPassword $securePass ` -ChangePasswordAtLogon $true -EmailAddress $emailAddr ` -Displayname "$FirstName $Lastname" -GivenName $Firstname ` -Surname $LastName -Enabled $true Write-Host "$LastName,$FirstName,$AccountName,$emailAddr,$password" } function Get-RandomPass{ $newPass = '' 1..10 | ForEach-Object { $newPass += [char](Get-Random -Minimum 48 -Maximum 122) } return $newPass }
How it works...
This script is composed of three functions: Create-Users
, Create-User
, and Get-RandomPass
. The first function starts by checking if a value was passed for the $fileName
parameter. If no value was included, it creates an array named $Users
and populates it with the number of test accounts defined by $numAccounts
. If $fileName
exists, it imports the target file as a CSV and populates the $Users
array.
Once the user list has been determined, each user account is cycled through. Additional account properties, such as the e-mail address and username, are populated based on user information, and if a password was not predefined, a random password is generated. Once the password is defined, then Create-User
is called.
The Create-User
function defines the $accountName
and $emailAddr
of the user account by combining various attributes. It then converts the password into a secure string that can be used when creating the user account. Lastly, New-ADUser
is called to create the user account in Active Directory with the defined user properties, and the user information is echoed to the console.
The third function named Get-RandomPass
uses a loop to create a 10 random characters, which are combined and returned as a random password. The function begins by creating a $newPass
variable as an empty string. The numbers 1
through 10
are passed into a ForEach-Object
loop that chooses a random character and appends it to the $newPass
variable. Once 10 characters have been added to the variable, the results are returned to whatever called the function.
Example output of creating multiple accounts is shown in the following screenshot:

There's more...
The following lists the additional features of AD users:
- Additional AD properties: The script as presented here sets only a minimum number of properties necessary for functional users. Several additional properties are as follows:
- Personal information, such as home address and phone numbers
- Organizational information, such as manager and office location
- Remote access settings
- AD information, such as home directory and logon script
- Workstations the user is allowed to log on to
- Template user: Instead of creating a new account for each user, it is possible to create a template account that is used for all new accounts. Template accounts are useful for maintaining common settings, such as logon script or home directory location, and then not keep the setting for all future accounts. To use a template account, simply load the account using
Get-ADUser
and reference the account using the–Instance
parameter. Refer the following example:$templateUser=Get-ADUser -Identity Administrator New-ADUser -Name Admin2 -Instance $templateUser -AccountPassword $securePass
See also
For a full list of available user properties that can be configured, see http://technet.microsoft.com/en-us/library/hh852238.