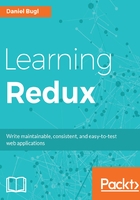
ES2015 - using destructuring and the rest operator to parse the action
For action parsing, ES2015 syntax comes in handy. We can easily and declaratively parse an object using destructuring.
We can manually pull out properties from the action object, as follows:
case CREATE_POST: {
const type = action.type
...
}
Instead of that, we can use destructuring, which would make the code look like this:
case CREATE_POST: {
const { type } = action
...
}
It is a bit like pattern matching in other languages—you specify what the object looks like after var/let/const, and it gets parsed/destructured into that form.
In combination with destructuring, you can also use default assignments, as follows:
const { type, user = 'anon' } = action
// instead of
const type = action.type
const user = action.user || 'anon'
In this example, if action.user is undefined, it will be set to 'anon'.
It is also possible to rename properties, as follows:
const { type: actionType } = action
// instead of
const actionType = action.type
The preceding code will store action.type in the actionType constant.
There is one more trick with destructuring—you can collect the rest of the properties into another object. This can be done with the rest operator. It works as follows:
const { type, ...post } = action
We can use the rest operator instead of manually collecting the rest of the properties:
const type = action.type
const post = {
user: action.user,
category: action.category,
text: action.text
}
This is pretty cool! Using the rest operator, we can save a lot of code and keep the post object extensible. For example, if we want to add a title to posts later, we could simply add a title property to the action and it would already be in the post object when using the rest operator.