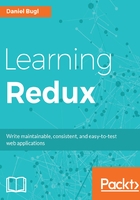
Handling CREATE_POST – creating a new post
Now that we know how to parse actions elegantly with ES2015 syntax, we can use that knowledge to parse the CREATE_POST action type:
First, we will pull out the data that we need from the action. In this case, we want to store everything—except for the type property—in a post object:
case CREATE_POST: {
const { type, ...post } = action
Then, we will insert this post object into our array and return the new state. To insert the post object, we use something similar to the rest operator—the spread operator, which is basically the reverse of the rest operator. Instead of collecting all properties of an object/elements of an array, it spreads them out:
return [ ...state, post ]
}
Using the spread operator achieves the same thing as using Array.prototype.concat(), which merges two arrays and returns a new one:
return state.concat([ post ])
That's it for the CREATE_POST action. As you can see, the ES2015 JavaScript syntax makes state changes very explicit and easy to write.