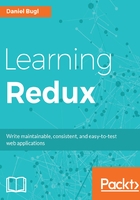
Functional components
To turn our little snippet into a React component, we need to turn the simple constant into a function. We will pass a name to the function and then output hello {name}!:
const Greeting = ({ name }) => ( <h1> hello {name}! </h1> )
Note how instead of passing name as a single argument, we are expecting an object with a name property to be passed. React passes all properties as an object via the first argument. Then we use de-structuring to pull out the name from the object. If we wanted to process the input before rendering JSX (for example, to make the name uppercase), we could do the following:
const Greeting = ({ name }) => { const uppercaseName = name.toUpperCase() return ( <h1> hello {uppercaseName}! </h1> ) }
While we could also put .toUpperCase() into the JSX expression, in most cases, it is much cleaner to carry out the processing outside of JSX.
Note that functional React components have to be pure. As a result, properties are read-only and cannot be modified. Furthermore, the same input will always result in the same output. Of course, applications are not static and sometimes values do need to change. You will learn how to deal with state later in this chapter.