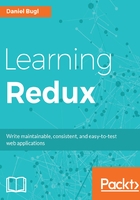
Using components
Now that we have a React component, we can render it via React.createElement. Putting everything together, our code should look as follows:
import React from 'react' import ReactDOM from 'react-dom' const Greeting = ({ name }) => { const uppercaseName = name.toUpperCase() return ( <h1> hello {uppercaseName}! </h1> ) } ReactDOM.render( React.createElement(Greeting, { name: 'dan' }), document.getElementById('root') )
Here is the great thing about React and JSX: instead of rendering simple HTML elements, we can render our React component, as if it was an HTML element:
ReactDOM.render( <Greeting name="dan" />, document.getElementById('root') )
Let's think about what happens here:
We call ReactDOM.render with our Greeting component and a name property. It will be rendered to the element with id="root", which we defined in our HTML template earlier.
The Greeting function gets called as follows: Greeting({ name: 'dan' }).
The Greeting function returns a simple HTML element: <h1>hello DAN!</h1>.
ReactDOM (re-)renders the simple HTML element into the element with id="root".
In our browser, we should now see a heading with the text "hello DAN!".
After learning how to define components and how to use them, you can put them together and reuse them to make a more complex application. The typical way to do this is to create an App component, which composes other components into the full application:
const App = () => (
<div>
<Greeting name="Daniel" />
<Greeting name="Destiny" />
</div>
)
Now, we can render the <App /> component instead of rendering the Greeting component directly:
ReactDOM.render( <App />, document.getElementById('root') )
Our application should now look as follows:
